Advanced#
Matplotlib an manim combined#
EDIT: This section was moved to https://manimplotlib.readthedocs.io/
[1]:
from manim import *
config.media_embed = True
Manim Community v0.17.3
Animations with OpenGL#
Just add the --renderer=opengl
flag, and animations will render with the OpenGL backend, which is still under development. Here are two examples: one for rendering a video (needs additional the --write_to_movie
flag) and one for a static 3D scene (including it)
[2]:
paramGL = "-v WARNING -s -ql --renderer=opengl --disable_caching --progress_bar None Example"
paramHGL = "-v WARNING -s -qh --renderer=opengl --disable_caching --progress_bar None Example"
parampGL = "-v WARNING -ql --renderer=opengl --write_to_movie --disable_caching --progress_bar None Example"
parampHGL = "-v WARNING -qh --renderer=opengl --write_to_movie --disable_caching --progress_bar None Example"
[3]:
%%time
%%manim $parampHGL
class Example(Scene):
def construct(self):
dot= Dot(color= YELLOW, radius=0.5)
self.play(dot.animate.shift(2*RIGHT).scale(2).set_color(BLUE))
self.wait()
CPU times: user 1.33 s, sys: 432 ms, total: 1.76 s
Wall time: 16.9 s
[4]:
%%time
%%manim $parampGL
class Example(ThreeDScene):
def construct(self):
resolution_fa = 42
self.set_camera_orientation(phi=75 * DEGREES, theta=-30 * DEGREES)
def param_gauss(u, v):
x = u
y = v
sigma, mu = 0.4, [0.0, 0.0]
d = np.linalg.norm(np.array([x - mu[0], y - mu[1]]))
z = np.exp(-(d ** 2 / (2.0 * sigma ** 2)))
return np.array([x, y, z])
gauss_plane = Surface(
param_gauss,
resolution=(resolution_fa, resolution_fa),
v_range=[-2, +2],
u_range=[-2, +2]
)
gauss_plane.scale(2, about_point=ORIGIN)
gauss_plane.set_style(fill_opacity=1,stroke_color=GREEN)
gauss_plane.set_fill_by_checkerboard(ORANGE, BLUE, opacity=0.5)
axes = ThreeDAxes()
self.add(axes,gauss_plane)
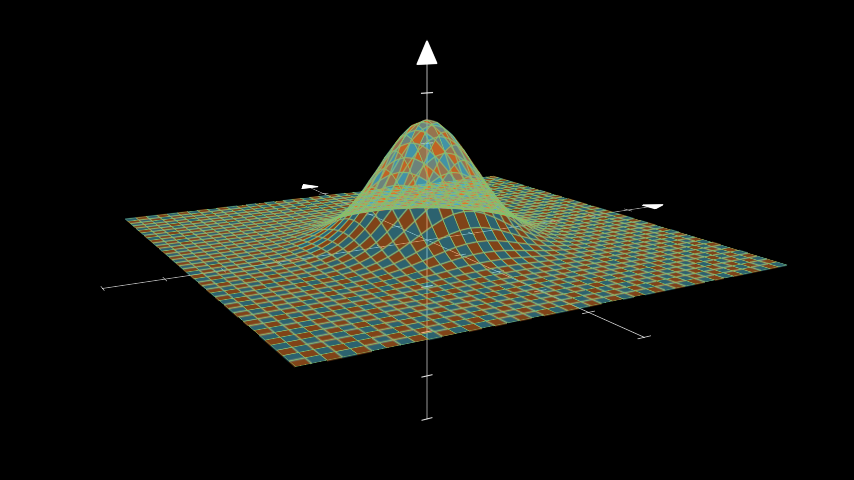
CPU times: user 3.83 s, sys: 99.7 ms, total: 3.93 s
Wall time: 3.78 s
[5]:
%%time
%%manim $param
## comparison of the same example to cairo:
class Example(ThreeDScene):
def construct(self):
resolution_fa = 42
self.set_camera_orientation(phi=75 * DEGREES, theta=-30 * DEGREES)
def param_gauss(u, v):
x = u
y = v
sigma, mu = 0.4, [0.0, 0.0]
d = np.linalg.norm(np.array([x - mu[0], y - mu[1]]))
z = np.exp(-(d ** 2 / (2.0 * sigma ** 2)))
return np.array([x, y, z])
gauss_plane = Surface(
param_gauss,
resolution=(resolution_fa, resolution_fa),
v_range=[-2, +2],
u_range=[-2, +2]
)
gauss_plane.scale(2, about_point=ORIGIN)
gauss_plane.set_style(fill_opacity=1,stroke_color=GREEN)
gauss_plane.set_fill_by_checkerboard(ORANGE, BLUE, opacity=0.5)
axes = ThreeDAxes()
self.add(axes,gauss_plane)
---------------------------------------------------------------------------
KeyError Traceback (most recent call last)
File <timed eval>:1
File ~/checkouts/readthedocs.org/user_builds/flyingframes/envs/latest/lib/python3.11/site-packages/IPython/core/interactiveshell.py:2478, in InteractiveShell.run_cell_magic(self, magic_name, line, cell)
2476 with self.builtin_trap:
2477 args = (magic_arg_s, cell)
-> 2478 result = fn(*args, **kwargs)
2480 # The code below prevents the output from being displayed
2481 # when using magics with decodator @output_can_be_silenced
2482 # when the last Python token in the expression is a ';'.
2483 if getattr(fn, magic.MAGIC_OUTPUT_CAN_BE_SILENCED, False):
File ~/checkouts/readthedocs.org/user_builds/flyingframes/envs/latest/lib/python3.11/site-packages/manim/utils/ipython_magic.py:139, in ManimMagic.manim(self, line, cell, local_ns)
136 renderer = OpenGLRenderer()
138 try:
--> 139 SceneClass = local_ns[config["scene_names"][0]]
140 scene = SceneClass(renderer=renderer)
141 scene.render()
KeyError: '$param'
[ ]: